0. 해당 글을 쓰게 된 배경
더보기
기존에는 c++을 통해서 코테를 준비하였다.
그러나, SSAFY를 시작하게 되면서 전공자의 경우는 Java로 모든 수업이 진행된다고 하였다.
어차피 Spring framework도 사용하고, 요즘 백엔드 직무 관련해서 많은 기업들에서 Java, node.js, python등으로 많이 보는 경향이 있기에 이참에 코테 언어를 변경해서 공부해야겠다고 마음먹었다.
따라서 코테용 주요 함수등을 정리해보면 나와 같은 상황에 있는 사람들에게 조금이나마 도움이 되지 않을까 싶어 글을 쓰게 되었다.
1. 필드의 구분
- 클래스 변수(static variable)
- 인스턴스 변수(instance variable)
- 지역 변수(local variable)
class Car {
static int modelOutput; // 클래스 변수
String modelName; // 인스턴스 변수
void method() {
int something = 10; // 지역 변수
}
}
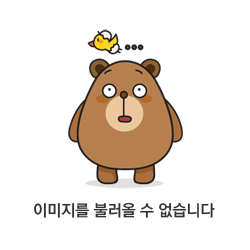
class Field {
static int classVar = 10; // 클래스 변수 선언
int instanceVar = 20; // 인스턴스 변수 선언
}
public class FieldEx2 {
public static void main(String[] args) {
int var = 30; // 지역 변수 선언
System.out.println("var = " + var); // 지역 변수 참조
Field myField1 = new Field(); // 인스턴스 생성
Field myField2 = new Field(); // 인스턴스 생성
System.out.println("Field.classVar = " + Field.classVar); // 클래스 변수 참조
System.out.println("myField1 = " + myField1.classVar);
System.out.println("myField2.classVar = " + myField2.classVar);
myField1.classVar = 100; // 클래스 변수의 값을 변경
System.out.println("Field.classVar = " + Field.classVar); // 클래스 변수 참조
System.out.println("myField1 = " + myField1.classVar);
System.out.println("myField2 = " + myField2.classVar);
myField1.instanceVar = 200;
System.out.println("myField1 = " + myField1.instanceVar); // 인스턴스 변수 참조
System.out.println("myField2 = " + myField2.instanceVar);
}
}
2. 메소드의 구분
- 클래스 메소드(static method)
- 인스턴스 메소드(instance method)
class Method {
int a = 10, b = 20;
// static int add() {return a + b;} // 다음과 같이 static 메소드는 메소드 내부에서 인스턴스 변수를 사용할 수 없다.
int add() {return a + b;}
static int add(int x, int y) {return x + y;}
}
public class TcpMethod2 {
public static void main(String[] args) {
System.out.println("Method.add(20, 30) = " + Method.add(20, 30));
Method myMethod = new Method();
System.out.println("myMethod.add() = " + myMethod.add());
}
}
3. 초기화 블록
- 명시적 초기화
- 생성자를 이용한 초기화
- 초기화 블록을 이용한 초기화
- 인스턴스 초기화 블록
- 인스턴스 초기화 블록은 단순히 중괄호({})만을 사용하여 정의
- 인스턴스 초기화 블록은 생성자와 마찬가지로 인스턴스가 생성될 때마다 실행
- 인스턴스 초기화 블록이 생성자보다 먼저 실행
- 여러 개의 생성자가 있으면 모든 생성자에서 공통으로 수행되어야 할 코드를 인스턴스 초기화 블록에 포함하여 코드의 중복을 막을 수 있다.
- 인스턴스 초기화 블록
class Car {
private String modelName;
private int modelYear;
private String color;
private int maxSpeed;
private int currentSpeed;
{ // 인스턴스 초기화 블록 : 단순히 중괄호({})만을 사용, 인스턴스가 생성될 때마다 실행, 인스턴스 초기화 블록이 생성자보다 먼저 실행
this.currentSpeed = 0;
}
Car() {}
public Car(String modelName, int modelYear, String color, int maxSpeed) {
this.modelName = modelName;
this.modelYear = modelYear;
this.color = color;
this.maxSpeed = maxSpeed;
}
public int getSpeed() {
return currentSpeed;
}
}
public class TcpMethod3 {
public static void main(String[] args) {
Car myCar = new Car();
System.out.println("myCar.getSpeed() = " + myCar.getSpeed());
}
}
2. 클래스 초기화 블록:
- 인스턴스 초기화 블록에 static 키워드를 추가하여 정의할 수 있다.
- 클래스가 처음으로 메모리에 로딩될 때 단 한 번만 실행됩니다.
class InitBlock {
static int classVar; // 클래스 변수
int instanceVar; // 인스턴스 변수
static { // 클래스 초기화 블록을 이용한 초기화
classVar = 10;
}
}
public class Member04 {
public static void main(String[] args) {
System.out.println("InitBlock.classVar = " + InitBlock.classVar); // 클래스 변수에 접근
}
}
4. 필드의 초기화 순서
- 클래스 변수: 기본값 -> 명시적 초기화 -> 클래스 초기화 블록
- 인스턴스 변수: 기본값 -> 명시적 초기화 -> 인스턴스 초기화 블록 -> 생성자
class InitBlock {
static int classVar = 10; // 클래스 변수의 명시적 초기화
int instanceVar = 10; // 인스턴스 변수의 명시적 초기화
static { // 클래스 초기화 블록을 이용한 초기화
classVar = 20;
}
{ // 인스턴스 초기화 블록을 이용한 초기화
instanceVar = 20;
}
public InitBlock() {
this.instanceVar = 30; // 생성자를 이용한 초기화
}
}
public class Member04 {
public static void main(String[] args) {
System.out.println("InitBlock.classVar = " + InitBlock.classVar); // 클래스 변수에 접근
InitBlock myInit = new InitBlock();
System.out.println("myInit.instanceVar = " + myInit.instanceVar);
}
}